Why Learn Spring MVC?
One of the most widely used frameworks for creating Java web applications is Spring MVC. By adhering to the Model-View-Controller (MVC) design, it makes creating enterprise-level apps easier. If you are a working professional looking to enhance your career or a student aiming to enter the IT industry, learning Spring MVC is a valuable investment.
In this blog, we will introduce you to Spring MVC, its key features, and why it is essential for modern web development.
What is Spring MVC?
Within the Spring Framework, Spring MVC (Model-View-Controller) is a potent framework that is frequently used to create web applications with Java. It offers an organized method for managing HTTP requests, business logic, and displaying views, which makes it easier to create scalable and reliable online applications.
According to the MVC paradigm, application logic is divided into three parts:
- Model: Shows the data and business logic of the application.
- View – Handles the presentation and UI logic.
- Controller – Manages user requests and directs them to the appropriate model and view.
With its flexibility, scalability, and integration capabilities, Spring MVC has become a go-to framework for Java developers aiming to build high-performance web applications.
Why Use Spring MVC?
Spring MVC offers several advantages that make it a preferred choice for Java developers and professionals:
- Separation of Concerns: The MVC pattern ensures a clear distinction between the model, view, and controller, making the application easy to manage and maintain.
- Simplicity of Integration: Spring MVC easily combines with other Spring modules, such as Spring Data, Spring Security, and Spring Boot.
- Powerful Annotation-Based Configuration: With @Controller, @RequestMapping, @RestController, and other annotations, developers can reduce XML configurations.
- Robust Exception Handling: Spring MVC provides centralized and flexible exception handling using @ExceptionHandler and @ControllerAdvice.
- Adaptable View Resolution: It works with a variety of view technologies, such as FreeMarker, Thymeleaf, and JSP.
- Testability: With dependency injection and the separation of concerns, unit testing and integration testing become easier.
Key Components of Spring MVC
To understand Spring MVC deeply, let’s break down its essential components:
1. DispatcherServlet
In a Spring MVC application, the Dispatcher Servlet serves as the front controller. Receiving incoming HTTP requests and assigning them to the proper handlers are its responsibilities.
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Bean
public DispatcherServlet dispatcherServlet() {
return new DispatcherServlet();
}
}
2. Controller
Controllers handle HTTP requests and define the business logic. They use @Controller or @RestController annotations.
@Controller
@RequestMapping(“/home”)
public class HomeController {
@GetMapping
public String home(Model model) {
model.addAttribute(“message”, “Welcome to Spring MVC!”);
return “home”; // View name
}
}
3. Model
The Model represents the application’s data. In Spring MVC, the Model interface is used to pass data to the view.
public class User {
private String name;
private int age;
// Getters and Setters
}
4. View
Presenting data to the user is the responsibility of the View layer. It can be implemented using JSP, Thymeleaf, or other templating engines.
<!– home.jsp –>
<html>
<body>
<h1>${message}</h1>
</body>
</html>
Spring MVC Request Handling Flow
Understanding the request flow in Spring MVC helps in designing better web applications:
1. An HTTP request is sent by the client.
2. After receiving the request, the dispatcher servlet forwards it to the relevant handler.
3. The controller responds after processing the request and interacting with the model.
4. The model gives the view the information it needs and refreshes the data.
5. View provides the client with the rendered answer.
Key Features of Spring MVC
- Loose Coupling – Components are loosely coupled, making development and maintenance easier.
- Annotation-Based Configuration – Uses annotations like @Controller, @RequestMapping, and @Autowired to simplify code.
- Multi-view technologies: like as JSP, Thymeleaf, and FreeMarker are supported via flexible view resolvers.
- Built-in Form Handling – Provides features for handling form submissions and validations.
- RESTful Web Services – Makes it easy to develop RESTful APIs.
- Exception Handling – Offers robust exception handling mechanisms.
- Integration with Other Technologies – Works well with Hibernate, JPA, and Spring Boot.
Commonly Used Spring MVC Annotations
Spring MVC provides a wide range of annotations to make development easier:
- @Controller – Defines a Spring MVC controller.
- @RestController – A specialized controller for REST APIs.
- @RequestMapping – Maps HTTP requests to controller methods.
- @GetMapping, @PostMapping, @PutMapping, @DeleteMapping – Handle specific HTTP methods.
- @RequestParam – Retrieves request parameters.
- @PathVariable – Retrieves values from the URL.
- @ModelAttribute – Binds form data to a model object.
- @ResponseBody – Converts method return values to JSON.
- @ExceptionHandler – Handles exceptions globally.
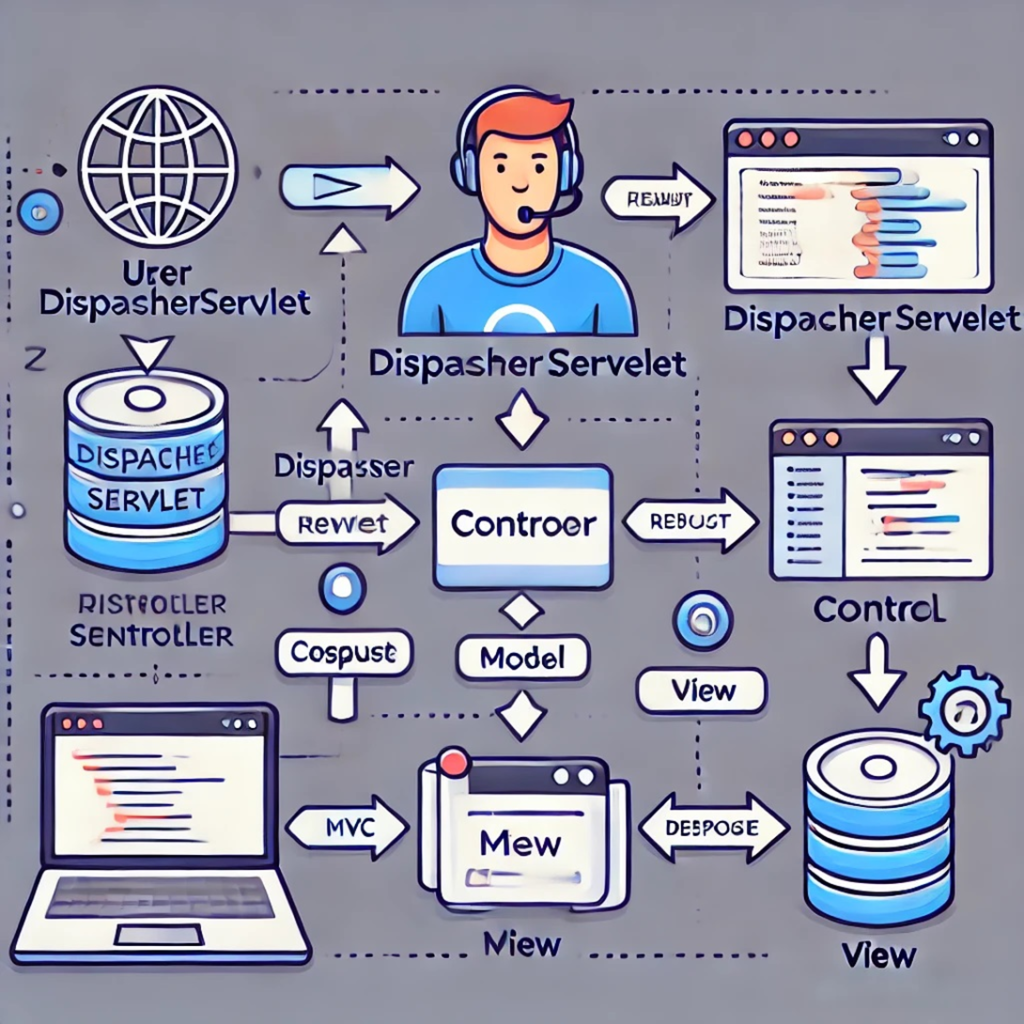
How Spring MVC Works: A Simple Flow
Let’s understand the request-processing flow in a Spring MVC application:
A request is sent by the user, and the Dispatcher Servlet receives it.
- Request Mapping – The DispatcherServlet matches the request with the correct controller method using @RequestMapping.
- Controller Processes the Request – The controller handles business logic and interacts with the model.
- View is Returned – The model data is passed to a view resolver, which renders the response.
- Response Sent to User: The user’s browser receives the created view back.
Simple Example: Creating a Basic Spring MVC Application
Below is a simple example of how to set up a basic Spring MVC controller.
@Controller
public class HelloController {
@RequestMapping(“/hello”)
public String sayHello(Model model) {
model.addAttribute(“message”, “Welcome to Spring MVC!”);
return “hello”; // Returns hello.jsp or hello.html
}
}
Explanation:
- @Controller – Marks the class as a Spring MVC controller.
- @RequestMapping(“/hello”) – Maps the request URL /hello to this method.
- Model – Holds data to be passed to the view.
- return “hello”; – Returns the view name (JSP or HTML page).
Spring MVC Market Statistics
- 80% of Enterprise Java Applications use Spring MVC as their web framework.
- 60% of Fortune 500 companies rely on Spring MVC for business applications.
- Over 50,000 job openings in India alone require Spring MVC expertise.
- Global Market Demand: Over the past three years, there has been a 25% increase in demand for Spring MVC developers.
Why Choose us for Spring MVC?
Learning Spring MVC from experts can significantly boost your career.
We offer:
- Hands-on Training – Work on real-world projects.
- Skilled Teachers: Gain knowledge from specialists in the field.
- Adaptable Learning Options: Both online and in-person instruction are offered.
- Job Assistance – Placement support for students and professionals.
- Affordable Fees – Best pricing for quality training.
Whether you are a student looking to build a career in Java development or a working professional aiming to upskill, our Spring MVC is perfect for you!
Conclusion
Spring MVC is an essential framework for Java developers building web applications. Its MVC architecture, annotation-based configuration, and seamless integration with other Spring modules make it a powerful choice for developing scalable and maintainable applications.
For students and professionals looking to master Spring MVC, practicing hands-on coding and exploring real-world projects is the best approach. To improve your development abilities, keep studying and staying current with new Spring features!