React is a popular JavaScript library used to create user interfaces. As such, it is a popular choice for web and mobile developers. If you’re looking for a job as a React developer, you’ll need to prepare for a range of React interview questions. In this article, we’ll cover some common React interview questions and answers.
WHAT IS REACT?
React is a JavaScript library that was created by Facebook and is used for building user interfaces (UIs). It is a component-based library, meaning that it is composed of individual components that can be reused, combined, and nested to create complex UIs. React is used to create single-page applications, mobile applications, and websites.
React is designed to be simple and easy to use, and it is designed to be declarative, meaning that the user interface is defined in a way that is easy to understand and maintain. This means that React is well-suited for developing complex UIs, such as those found in enterprise applications. React is also a component-based library, meaning that it is composed of individual components that can be reused, combined, and nested to create complex UIs.
React is also designed to be highly performant, meaning that it can handle large amounts of data and render it quickly. This is made possible by the use of a virtual DOM, which is a JavaScript representation of the actual DOM (Document Object Model). The virtual DOM is used to track changes to the DOM, and React can then efficiently update the DOM with only the necessary changes.
React is also highly extensible, meaning that it can be extended with additional libraries and frameworks. This allows developers to create complex applications using React components, such as Redux for state management and React Router for routing.
Finally, React is open-source, meaning that it is free and open to anyone who wishes to use it. As such, it has become one of the most popular JavaScript libraries, and it is used by many companies and organizations, including Facebook, Airbnb, and Netflix.
In conclusion, React is a JavaScript library that is used for building user interfaces. It is component-based, meaning that it is composed of individual components that can be reused, combined, and nested to create complex UIs. It is also designed to be simple and easy to use, highly performant, and highly extensible. Finally, it is open-source and is used by many companies and organizations.
WHAT ARE THE ADVANTAGES OF USING REACT?
React is a popular JavaScript library created by Facebook used for building user interfaces. It was released in 2013 and since then it has become one of the most widely used JavaScript libraries for developing web applications. React allows developers to build powerful, interactive, and fast user interfaces for web applications.
In this article, we’ll go over some of the advantages of using React.
1. Easy to Learn and Use
React is relatively simple to learn and use compared to other JavaScript libraries. It is based on JavaScript, which is a popular language, so most developers are already familiar with it. The syntax is also straightforward and easy to understand. This makes it easier for developers to write React code and understand how it works.
2. Component-Based Structure
React uses a component-based structure, which makes it easier to develop large-scale applications. Components are autonomous, reusable segments of code. This makes it easier to maintain code and reuse components in different parts of an application.
3. Virtual DOM
React uses a Virtual DOM (Document Object Model) to render components faster. The actual DOM is represented by the Virtual DOM in JavaScript. It is used to diff the previous version of the DOM and the new version of the DOM, so only the necessary changes are made. This makes it much faster than traditional DOM manipulation.
4. One-Way Data Flow
React uses a one-way data flow, which makes it easier to reason about and debug applications. Data is passed down from a parent component to a child component, and changes are made only in the parent component. This makes it easier to keep track of data and ensure that components are always in sync.
5. Testability
React components are easy to test, which makes it easier to ensure that they are working correctly. React components can be tested using tools like Enzyme and Jest. This makes it easier to make sure that components are working correctly and can scale with the application.
These are just some of the advantages of using React. React is an increasingly popular library for developing web applications and these advantages make it an attractive choice for developers.
WHAT IS THE DIFFERENCE BETWEEN PROPS AND STATE IN REACT?
In React, props and state are two core concepts that are used to handle data in a React application. Props and state both represent changes in the UI, but they differ in how they are used and when they are used.
Data is transferred between components using props. A parent component can give data to its child components using props. Props are read-only, which means that the parent component is responsible for setting the props, but the child component cannot change the props.
State, on the other hand, is used to store data within a component. State is mutable, which means that the component can change its own state. State allows a component to keep track of its own data and update itself when necessary.
In summary, props are used to pass data from one component to another, while state is used to store data within a component. Props are read-only, while state is mutable. Props are set by the parent component, while state is set and managed by the component itself.
Are you guys willing to acquire ReactJS skills, then ProIT Academy is coming up with their Best React JS Classes in Pune where you can master the skills.
HOW DO YOU CREATE A COMPONENT IN REACT?
Creating a component in React is a very straightforward process, and it is essential to understand the fundamentals of the React library before attempting to build components. A React component is a reusable piece of code that is responsible for rendering a specific part of the UI. It is responsible for displaying the data, managing state, and providing a declarative way of defining UI elements.
To create a component in React, the first step is to create a JavaScript class that extends the React component class. This is done by using the React.createClass method. This class is used to define the structure and behaviour of the component. The render method is used to define the HTML that should be rendered when the component is used in the application.
The next step is to create a constructor for the component class. The constructor is used to define the initial state of the component and to bind event handlers to the component. This allows the component to respond to user input and update the state accordingly.
Once the component is initialized, the next step is to add the render method. This method is responsible for returning the HTML that should be rendered when the component is used in the application. The render method can be used to define the UI elements that should be rendered and how they should be styled. Styling can be done using inline styles or by using a separate CSS file.
The last step is to create a render function. The render function is responsible for taking the data from the component’s state and displaying it on the page. This is done using the ReactDOM.render method. It takes two arguments, the component instance and the element where the component should be rendered.
Creating a component in React is a very simple process that can be completed in a short amount of time. It is a great way to keep applications modular and organized, and it allows developers to easily make changes to the application without having to rewrite the entire codebase. By following the steps outlined above, developers can quickly create components and add them to their application.
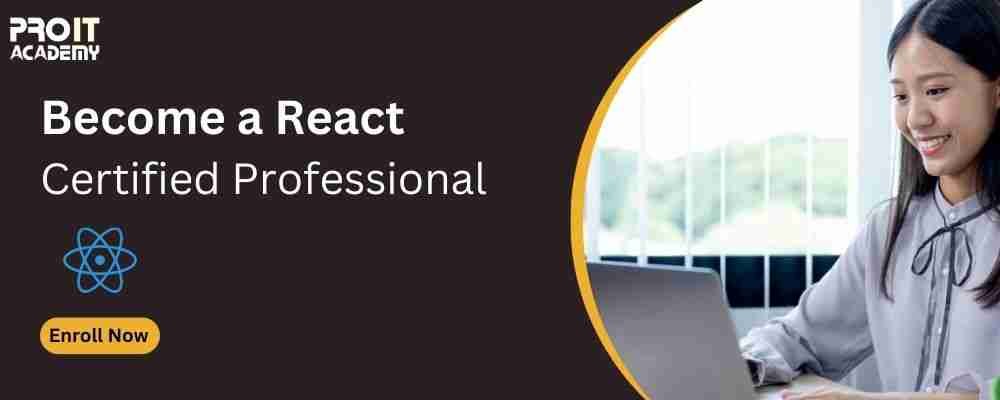
WHAT IS THE DIFFERENCE BETWEEN A CLASS COMPONENT AND A FUNCTIONAL COMPONENT IN REACT?
React components are the building blocks of a React application and can be either class components or functional components. While both types of components offer the same end result, they are implemented differently and have different use cases.
Classes in JavaScript called class components extend the React Component class. They are used when a component needs to have its own state or contain complex logic. They have the added benefit of being able to use lifecycle methods such as ComponentDid Mount and ComponentDid Update.
Functional components, on the other hand, are JavaScript functions. They are used when a component does not need to have its own state or contain complex logic. They are simpler to write than class components and are often used for presentational components.
The main difference between class components and functional components is that class components can have state and lifecycle methods while functional components do not. Class components also have access to the keyword, which allows them to access props and state from within the component. Functional components do not have access to the keyword, so they must use the props argument to access props.
Class components are the preferred choice for most React applications since they can contain state and lifecycle methods. However, functional components are great for simpler components where state and lifecycle methods are not needed. In conclusion, the main difference between class components and functional components is that class components can have state and lifecycle methods while functional components do not. Depending on the complexity of your component, one type may be more suitable than the other.
WHAT THE LIFE CYCLE METHODS ARE IN REACT?
Lifecycle methods are methods that are called at specific points in a components lifecycle. They are an important part of React and are used to help keep your components up to date and to provide additional functionality.
React components have several lifecycle methods that can be used to monitor and update the state of the component. These methods are called when the component is created, updated, and destroyed. Each lifecycle method is triggered at the appropriate time and provides an opportunity to perform logic or modify state.
The most commonly used lifecycle methods are:
1. Component Did Mount: This method is called after the component is mounted to the DOM and any initializations that need to be done can be done here. It is also a good place to make any AJAX calls or set up subscriptions.
2. Component Did Update: This method is called after the component has been updated and is used to perform any updates that need to be done. It is also a good place to make any AJAX calls or set up subscriptions.
3. Component Will Unmounts: This method is called before the component is unmounted from the DOM and is used to perform any clean up that needs to be done.
4. ComponentWillReceive Props: This method is called before a component receives new props and is used to update the state of the component based on the new props that are being passed in.
5. Should Component Update: This method is called before the component is re-rendered and is used to determine if the component should be re-rendered or not. This can be used to optimize performance by avoiding unnecessary re-renders.
These are the most commonly used lifecycle methods but there are several other lifecycle methods that can be used to monitor and update the state of the component. Understanding how and when to use these lifecycle methods is an important part of developing react applications.
Lifecycle methods are an important part of React and are used to help keep your components up to date and to provide additional functionality. By understanding when and how to use lifecycle methods you can ensure that your React applications are running optimally and are providing the best user experience.

Book Your Time-slot for Counselling !
WHAT IS THE PURPOSE OF THE RENDER METHOD IN REACT?
The render method in React is one of the most important concepts of React and is a vital part of the React component lifecycle. The render method is the only required method in a React Component, and it’s used to render the React element into the DOM. It’s the method that takes in the components props and state and returns a React element.
The primary purpose of the render method is to create and return a React element that represents the UI of the component. The React element can be a single HTML element, a set of elements, or a combination of both. It can also be a React component. The React element created by the render method is what gets displayed to the user.
The render method is also responsible for updating the UI when the component’s state or props change. When the state or props of a React component change, the render method gets called again. This is called reconciliation. The render method takes the new state and props and creates a new React element. This new element is then compared to the previous element, and the difference is used to update the DOM.
In addition to creating and updating the UI, the render method is also responsible for triggering side effects. Side effects are actions like network requests, setting up event listeners, or subscribing to state changes. These side effects are triggered before or after the render method is called, depending on the type of side effect.
The render method is the most important part of a React component, and it’s what makes React so powerful. It’s responsible for creating and updating the UI, and it’s also responsible for triggering side effects. Without the render method, React components would be unable to work properly.
The render method is also the entry point of a React component. It’s the first method that’s called when a React component is initialized. From there, the other methods in the component are called to set up the component’s state, trigger side effects, and update the UI.
WHAT IS THE PURPOSE OF THE COMPONENT DID MOUNT METHOD IN REACT?
The Component Did Mount method is a lifecycle method in React that is called after a component is mounted to the DOM. This method is a good place to set up any subscriptions or any other initialization tasks that need to occur once the component has been mounted. It is important to note that any set up that requires DOM nodes should occur in this method, as the component will not have access to the DOM until after the mount has been completed.
When a React component is created, the Component Did Mount method is the first lifecycle method that is called. This method is called after the initial render of the component, allowing the component to have access to the DOM and any other properties that are necessary for the component to function properly. This method is often used to make API calls, set up subscriptions, or perform any other initialization tasks that need to occur once the component is mounted to the DOM.
One of the key benefits to using the Component Did Mount method is that it allows developers to create components that are self-contained and have an independent life cycle. This means that the component is not dependent on any other components and can be used without the need for any outside dependencies. By utilizing the Component Did Mount method, developers can ensure that the component is set up correctly and running properly before any other tasks need to be performed.
In addition, the ComponentDid Mount method is also a great place to perform any data fetching or other operations that may need to be done before the component is rendered. This allows the component to be rendered quickly and without delay. It also ensures that any data that is required is available when the component is rendered, allowing the component to function correctly.
Finally, the Component Did Mount method is also a great place to set up any subscriptions or other tasks that need to be completed once the component is mounted. This ensures that the component is able to receive any updates that may occur once the component is mounted and that any necessary actions can be taken as soon as the updates occur.
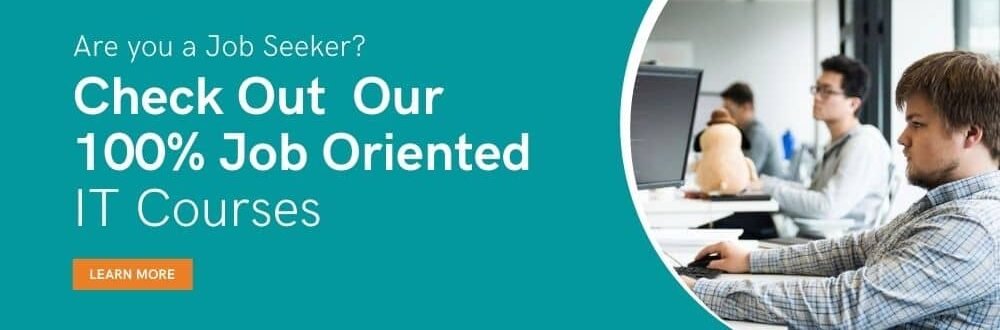
WHAT IS THE DIFFERENCE BETWEEN A CONTROLLED COMPONENT AND AN UNCONTTROLLED COMPONENT IN REACT?
React provides an efficient way to keep track of the state of an application and render components based on that state. Components are the building blocks of React, and understanding the differences between controlled and uncontrolled components is essential for developers looking to create a robust, user-friendly application.
A controlled component is a React component that is “controlled” by the application’s state. This means that the state of the component is managed by the parent component and is not modified directly by the user. For example, when a user clicks a button, the parent component updates the state and the child component re-renders with the updated state. This is an example of a controlled component.
An uncontrolled component, on the other hand, is a React component that is not “controlled” by the application’s state. Instead, the user is in charge of the state of the component and can modify it directly. For example, if a user enters text into an input box, the input box will update its value with the text the user has entered. That’s an example of an uncontrolled component.
The main difference between a controlled and uncontrolled component is how the component’s state is managed. In a controlled component, the state is managed by the parent component, while in an uncontrolled component, the state is managed by the user. Another difference is that controlled components are typically easier to maintain, since the state is managed by the parent component. However, uncontrolled components provide more flexibility, since the user is in control of the state.
Finally, it is important to note that there are some cases where using an uncontrolled component is the best option. For example, if the user needs to enter a large amount of text, it might make more sense to use an uncontrolled component rather than a controlled one.
WHAT IS THE DIFFERENCE BETWEEN AN ELEMENT AND A COMPONENT IN REACT?
The two main building blocks of a React application are elements and components. Both elements and components are integral parts of React, but there are some important differences between them.
An element is a plain JavaScript object that describes what should be displayed on the screen. It is a building block of a React application and is used to create a tree of React components that will eventually be rendered to the DOM. An element is a basic unit of the React UI and is described by a “props” object. The props object contains information about an element including its type, its attributes, and its children.
A component is a more advanced version of an element. It is a piece of code that describes how a UI element should behave and look. It is a function or class that is used to extend the capabilities of the element. It can be used to add additional features and functionality to an element, such as state and lifecycle methods.
The main difference between elements and components is that elements are concerned with the UI and components are concerned with the behaviour. Elements are responsible for displaying the UI on the screen, while components are responsible for controlling the behaviour of the UI.
Elements are also referred to as “stateless components” because they don’t have any internal state to manage. They are immutable and cannot be changed after they are created. Components, on the other hand, are “stateful components” because they maintain their own internal state.
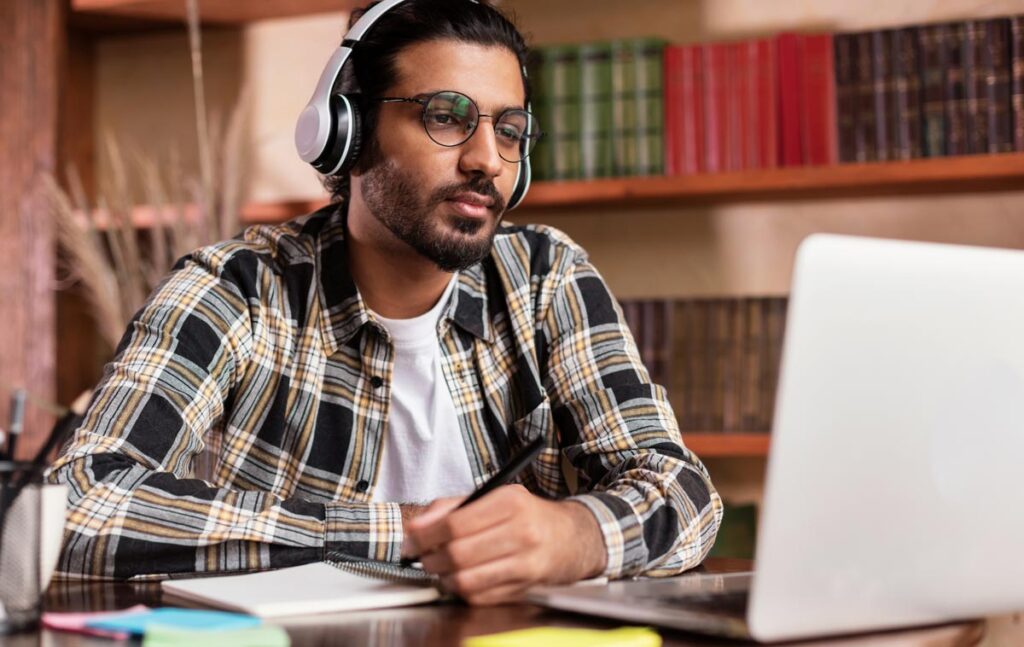
Do you need help to create your career path ?
WHAT IS THE PRUPOSE OF THE USE STATE HOOK IN REACT?
The purpose of the useState hook is to allow developers to create components that can maintain their own state. In React, state is an object that stores data that a component needs to render correctly. By using the useState hook, developers can easily keep track of this data, and make sure it is up to date.
The useState hook is an important part of React because it allows developers to easily manage the state of their components. Without the useState hook, developers would need to manually manage the state of their components, which would be a tedious and time-consuming task.
The useState hook also allows developers to create components that are more reusable. When a component is written in a way that uses the useState hook, it can be used in multiple places with different sets of data. This makes it easier for developers to reuse components, and makes their code more efficient.
Another benefit of the useState hook is that it allows developers to make their components more dynamic. For example, a component can be written in a way that it can update its state in response to user interactions or external data. This makes it easier for developers to create components that are interactive and responsive.
Finally, the useState hook allows developers to write components that are easier to debug. This is because components written in a way that uses the useState hook are easier to understand, as each piece of state is clearly defined. This makes it easier for developers to find and fix errors in their code.
Check out Job Oriented Courses for a brighter and promising future.
WHAT THE PURPOSES OF THE USE EFFECT HOOK IS IN REACT?
The useEffect hook in React is a powerful feature of React that allows developers to access lifecycle methods and state changes within a functional component. It allows developers to execute code based on certain conditions, such as when a component is mounted or unmounted or when the component’s props or state has changed.
The useEffect hook is a way for developers to access lifecycle methods and state changes within a functional component. It allows developers to execute code based on certain conditions, such as when a component is mounted or unmounted or when the component’s props or state has changed.
The useEffect hook is often used to manage side effects, such as fetching data or running animations. It is also commonly used to update the UI based on state changes.
One of the main benefits of the useEffect hook is that it allows developers to write code that is more declarative and easier to read and understand. It also allows developers to separate logic and side effects from the main component.
The useEffect hook can be used in several different ways, depending on the needs of the developer. For example, it can be used to perform an action when a component mounts, such as fetching data or running an animation. It can also be used to perform an action after a state update, such as updating the UI or running a different animation.
The useEffect hook is a powerful feature of React that allows developers to access lifecycle methods and state changes within a functional component. It allows developers to execute code based on certain conditions, such as when a component is mounted or unmounted or when the component’s props or state has changed. This makes it easier for developers to write code that is more declarative and easier to read and understand.